What is Docker and how to use it?
Docker is a software framework used for packaging, running, deploying and managing multiple apps on a server. Docker delivers OS-level virtualization to package a software called a container. It's an open-source and the most popular containerization platform.
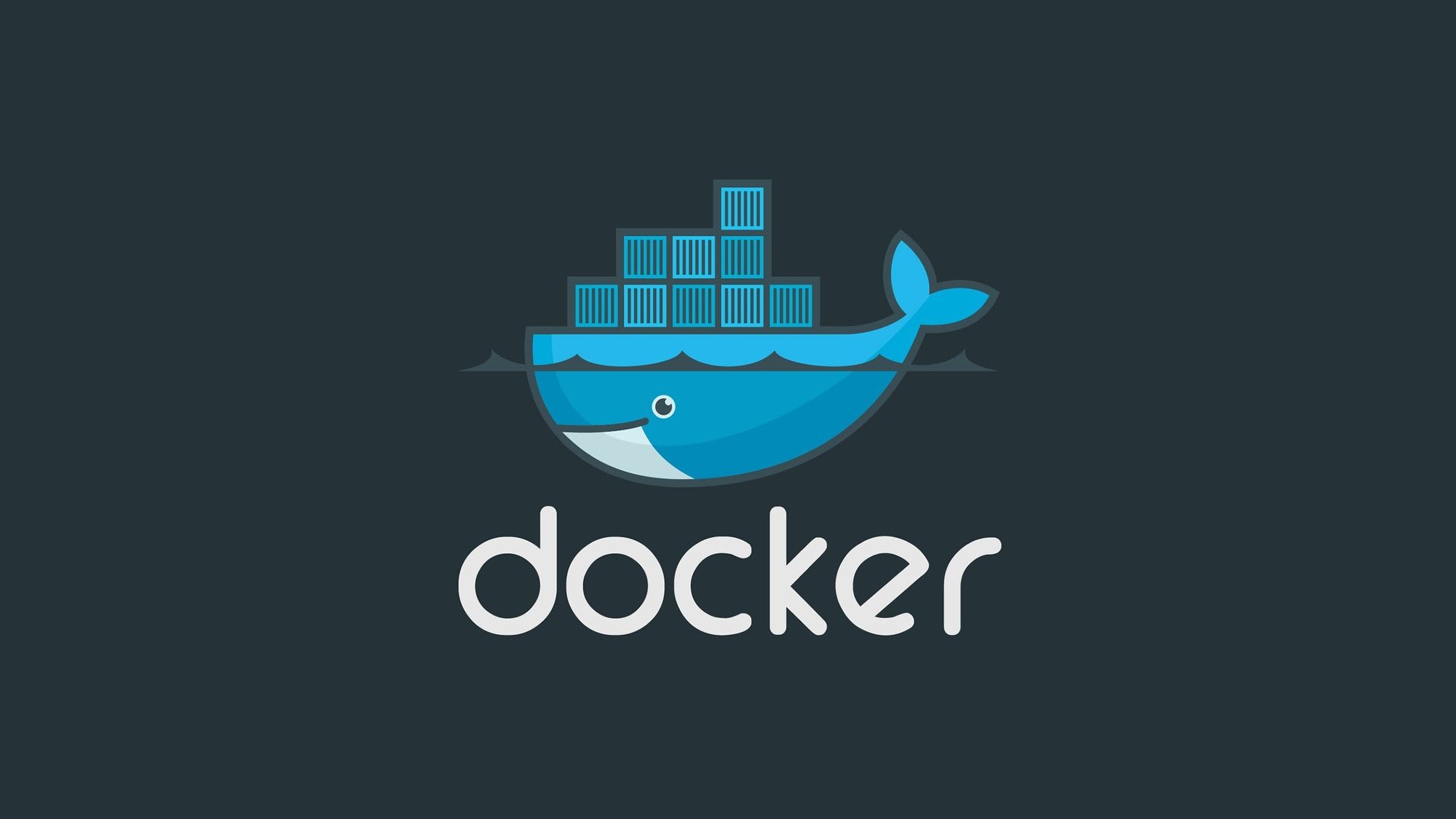
Docker
Docker is a software framework used for packaging, running, deploying and managing multiple apps on a server. Docker delivers OS-level virtualization to package a software called a container. It's an open-source and the most popular containerization platform.
With docker, we can package our application for a specific environment. For example, our app needs:
- Node 16.15
- MongoDB 5.0.1
- App
Docker got you covered. You can package your app and run it on any machine that can run docker.
Why docker is required ?
Docker was developed to solve a very common problem in a software development environment "It work's on my machine" there are a lot of cases a developer would say:

Docker Image
Image is a read-only template/snapshot for docker that contains instructions to run the docker container. Docker image also includes server environment configuration already packed. We can also build our custom image on the top of Base-OS Image. Alpine, Ubuntu, NodeJS and Python are a few examples of Base Images.
Docker Image is then hosted on a docker registry. One of them is DockerHub (Which is docker’s official registry hosting provider). Other registry providers are Amazon Elastic Container Registry (ECR)
Any developer can then pull the image (the app) with docker pull
and run that in a docker container, or build another image on top of it. It is important to remember that 1 docker image can be used or run in multiple Docker containers.
Example
Let’s run a sample docker image hello-world hosted on a Dockerhub:
docker run hello-world
The response would be something like this
Unable to find image 'hello-world:latest' locally
latest: Pulling from library/hello-world
2db29710123e: Pull complete
Digest: sha256:80f31da1ac7b312ba29d65080fddf797dd76acfb870e677f390d5acba9741b17
Status: Downloaded newer image for hello-world:latest
Hello from Docker!
This message shows that your installation appears to be working correctly.
Sample pre-build docker image.
Redis
docker run -d -p 6379:6379 --name redis redis
Postgres
docker run --rm -it -p 5432:5432 -e POSTGRES_PASSWORD=mydb_secret_password -e POSTGRES_USER=postgres -e POSTGRES_DB=InitialDB postgres
Docker container flags
-p
flag is mapping host port with docker port for port forwarding like-p host:container
-e
flag is specifying environment variables and their values like-e key=value
Docker commands:
To view a list of images downloaded on your local machine, docker image ls
would help with a response like:
docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
node latest 4c60c47ec5e4 1 hours ago 996MB
nginx latest 0e901e68141f 7 hours ago 142MB
memcached latest 7665ebc98caa 8 hours ago 89.2MB
postgres latest dd21862d2f49 10 days ago 376MB
redis latest 87c26977fd90 2 months ago 113MB
To remove a downloaded image with image id.
docker rmi 4c60c47ec5e4
Docker Container
Docker Container is like a process running on Operating System which uses a docker image. With Docker Container, you can run hundreds of apps with different versions in a completely isolated environment on the same machine without the need for any hypervisor or a guest OS.
For example:
Imagine, there are two NodeJS apps
- appA works with Node 14 and requires a database of Postgres 12
- appB is developed for Node 16 and requires Postgres 14 version respectively.

Docker Container Architecture
Difference between Docker Container & virtualized OS
Container | Virtual Machine |
---|---|
A complete isolated environment for an APP. | An Abstraction of machine resources. (Physical Hardware) |
Docker is required to manage multiple container, but all the containers run on a same Host OS. | Hypervisor like VirtualBox, VMware is required to do OS-Level virtualization and manages those virtual machine. |
Allow running multiple apps on a single machine in an isolated environment, without the need of any other guest OS. | Each virtual machine needs a full flugged Guest OS like Windows, Ubuntu to run the app. Which may require a license to use. |
Quick start, more lightweight | Slow to start and resource intensive |
All the configuration is done once and shared in a Dockerfile or docker-compose | Requires a lot of configurations to be done |
It is also crucial to know that: when you stop the container, any state or data changed or created inside the container will be lost and reset to the image's default state. There can situations where you are required to store the data persistently on a local machine, which can be shared by multiple containers. Here comes Docker Volume to rescue!
Docker Volumes
Docker volume is a dedicated shared folder on a host machine. It is used as persistent storage for Docker Container apart from the container itself, which could be managed and maintained by the Docker engine.
As we discussed, a dockerized container is a special kind of process and the data created inside the container vanishes when the container turns off. But, we can attach a volume with the container that acts as persistent data storage for the container and files created inside a container could stick around.
Commands
To list of all of the docker volumes in your local machine.
docker volume ls
DRIVER VOLUME NAME
local app-volume
local another-app-volume
To view more information on a specific volume, we would type:
docker volume inspect app-volume
The response would be something like this:
[
{
"CreatedAt": "2022-05-17T12:00:51Z",
"Driver": "local",
"Labels": null,
"Mountpoint": "/var/lib/docker/volumes/app-volume/_data",
"Name": "app-volume",
"Options": null,
"Scope": "local"
}
]
Docker CLI
- Docker Desktop
Docker Desktop is available for almost all the popular OS (macOS, Linux and Windows)

Dockerize an App with Dockerfile
The process of packaging an app using a file name Dockerfile
is known as Dockerizing.
A Dockerfile is like a blueprint for building docker image.
FROM alpine:latest
RUN apk add --update htop && rm -rf /var/cache/apk/*
CMD ["htop"]
Dockerfile Commands
ARG | ARG used to define a variable that can be used at the build time inside the Dockerfile |
RUN | Execute the command inside the docker image building process on the top of the current image. |
COPY | Copy files from your current working directory to docker image. |
ENV | Used to specify environment variables like port, and directory for the docker image. ENV Variables will be available at build time and at execution of container as well. |
EXPOSE | Exposing port(s) of a docker container, which can be mapped from container to local machine using port forwarding. |
CMD | There will be only one cmd in Dockerfile , that actually tells the container how to run the app. |
ENTRYPOINT |
Ignore a file with .dockerignore
File named .dockerignore
works exactly the same as .gitignore
or .npmignore
node_modules/ # node_module build
.env # virtual environment variables
.venv
venv/ # virtual environment of python app
build/ # python build
.git/ # current project git repository information
logs/ # Any logs
*.log
*.md # Markdown readme file
Build docker image
It is recommended that you setup a username on Dockerhub before name a build image.
docker build -t [username]/[image-name]:[tag] .
docker build -t saqe/sample-docker:1.0 .
By default the tag of the docker image would be :latest

To view that image.
docker image ls
REPOSITORY TAG IMAGE ID CREATED SIZE
saqe/sample-docker 1.0 c225b3a4c6ed 7 minutes ago 6.43MB
To start the saqe/sample-docker:1.0 into a container.
docker run -t saqe/sample-docker:1.0

Docker Compose
A docker-compose.yml
will look like this:
services:
web:
build: .
ports:
- "8000:5000"
volumes:
- .:/code
- logvolume01:/var/log
links:
- redis
redis:
image: redis
volumes:
logvolume01: {}
docker-compose up
Docker CLI Commands
docker ps
Docker processdocker run
Create and start the containerdocker create
Create containerdocker exec
Run commands in container for oncedocker volume
Create a docker volume.docker network
Create a docker network.docker rm
Remove container.docker images
List the images.docker rmi
Remove image.docker build
Build a new image fromDockerfile
.docker push
Push your image to docker repo.docker pull
Download an image from docker repo.docker commit
Create an image from container.
Learning Docker
https://labs.play-with-docker.com
Kubernetes
Kubernetes also know as "K8s" is a container orchestration application to manage cluster of hosts/containers. Kubernetes automates software deployment, scaling and management.
Kubernetes was originally developed by Google, but now actively maintained by Cloud Native Computing Foundation.
Q: What is Kubernetes?✳️
— Satyen Kumar (@SatyenKumar) February 24, 2022
Kubernetes is a word which appears during the interview and disappears after the candidate joining!